Libraries for DataScience
Introduction
Data Science is a multidisciplinary field that uses scientific methods, processes, algorithms, and systems to extract knowledge and insights from structured and unstructured data. In this tutorial, we will cover some of the most important libraries used in the field of Data Science, including their functionalities and examples of how to use them.
NumPy
NumPy (Numerical Python) is a fundamental package for scientific computing in Python. It provides support for arrays, matrices, and many mathematical functions.
Example
First, install NumPy using pip:
Example code:
import numpy as np # Create an array array = np.array([1, 2, 3, 4, 5]) print("Array:", array) # Perform basic operations print("Mean:", np.mean(array)) print("Median:", np.median(array)) print("Standard Deviation:", np.std(array))
Output:
Array: [1 2 3 4 5] Mean: 3.0 Median: 3.0 Standard Deviation: 1.4142135623730951
Pandas
Pandas is a powerful, open-source data manipulation and analysis library for Python. It provides data structures and functions needed to manipulate tables and time series data.
Example
First, install Pandas using pip:
Example code:
import pandas as pd # Create a DataFrame data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [24, 27, 22], 'City': ['New York', 'Los Angeles', 'Chicago'] } df = pd.DataFrame(data) print("DataFrame:") print(df)
Output:
DataFrame: Name Age City 0 Alice 24 New York 1 Bob 27 Los Angeles 2 Charlie 22 Chicago
Matplotlib
Matplotlib is a plotting library for the Python programming language and its numerical mathematics extension NumPy. It provides an object-oriented API for embedding plots into applications.
Example
First, install Matplotlib using pip:
Example code:
import matplotlib.pyplot as plt # Create a simple plot x = [1, 2, 3, 4, 5] y = [2, 3, 5, 7, 11] plt.plot(x, y) plt.title('Simple Plot') plt.xlabel('x-axis') plt.ylabel('y-axis') plt.show()
Output:
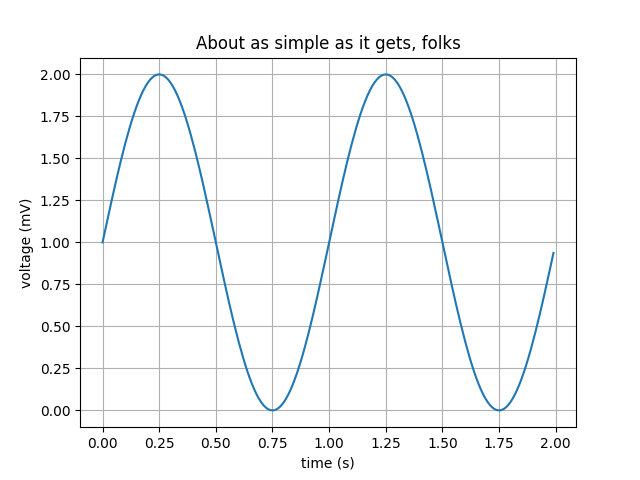
Scikit-Learn
Scikit-Learn is a machine learning library in Python that provides simple and efficient tools for data mining and data analysis. It is built on NumPy, SciPy, and Matplotlib.
Example
First, install Scikit-Learn using pip:
Example code:
from sklearn import datasets from sklearn.model_selection import train_test_split from sklearn.linear_model import LogisticRegression from sklearn.metrics import accuracy_score # Load dataset iris = datasets.load_iris() X = iris.data y = iris.target # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42) # Create a logistic regression model model = LogisticRegression(max_iter=200) model.fit(X_train, y_train) # Make predictions y_pred = model.predict(X_test) # Calculate accuracy accuracy = accuracy_score(y_test, y_pred) print("Accuracy:", accuracy)
Output:
Accuracy: 1.0
TensorFlow
TensorFlow is an end-to-end open-source platform for machine learning developed by Google. It has a comprehensive, flexible ecosystem of tools, libraries, and community resources that lets researchers push the state-of-the-art in ML, and developers easily build and deploy ML-powered applications.
Example
First, install TensorFlow using pip:
Example code:
import tensorflow as tf # Create a constant tensor hello = tf.constant('Hello, TensorFlow!') # Start a TensorFlow session sess = tf.Session() # Run the session print(sess.run(hello))
Output:
b'Hello, TensorFlow!'